Quick Start Guide
How to install the Fingerprint Platform in your application.
Fingerprint is a device intelligence platform offering device identification with 99.5% accuracy. Protect your application from fraud, spam, account takeover, bots, and other malicious activity with fingerprinting as a service.
Create an Account
Sign up for your free trial to get started, no credit card is required.
If you have network issues accessing our dashboard, check out this troubleshooting page.
Get your API Key
- In the Dashboard, navigate to App Settings in the left-hand menu, and then click API Keys.
- Under Public API Keys, copy or save your public API key to run the examples below.
Install the client-side Agent
Once you've created your account and got your public API key, you can install the JavaScript agent on your website or a native agent in your mobile application. The agent collects browser or device attributes, sends them to the Fingerprint Platform, and receives a visitor ID for that browser or mobile device. For simplicity, we refer to the client-side agent as "JS agent" further in this guide.
The easiest way to install the agent is by going to App settings > Integrations and picking your preferred web or mobile library.
For example, you can add the JS agent directly into your HTML using our CDN or install it as an NPM package:
npm install @fingerprintjs/fingerprintjs-pro
yarn add @fingerprintjs/fingerprintjs-pro
Add the JavaScript snippet below to all pages where you want to identify visitors:
<script>
// Initialize the agent at application startup.
// Some ad blockers or browsers will block Fingerprint CDN URL.
// To fix this, please use the NPM package instead.
const fpPromise = import('https://fpjscdn.net/v3/<<browserToken>>')
.then(FingerprintJS => FingerprintJS.load());
// Get the visitor identifier when you need it.
fpPromise
.then(fp => fp.get())
.then(result => console.log(result.visitorId));
</script>
import FingerprintJS from '@fingerprintjs/fingerprintjs-pro'
// Initialize an agent at application startup.
const fpPromise = FingerprintJS.load({ apiKey: '<<browserToken>>' })
// Get the visitor identifier when you need it.
fpPromise
.then(fp => fp.get())
.then(result => console.log(result.visitorId))
Every identification request will return a unique visitorId value for the current visitor. The visitor ID accuracy is 99.5% — out of 1,000 random unique visitors, up to 5 identifiers may be incorrect.
For more information, see the JS Agent API Reference. If you need to identify users on a mobile platform instead, see the Android or iOS guide.
EU and Asia Region users
If you chose the EU or Asia region during registration, please add region
to the JS agent initialization:
FingerprintJS.load({
apiKey: '<<browserToken>>',
+ region: 'eu'
})
FingerprintJS.load({
apiKey: '<<browserToken>>',
+ region: 'ap'
})
This will guarantee that your data will always be kept in the chosen region and will not be replicated in other data centers.
Avoiding ad blockers and privacy-focused browsers
Requests from the JS Agent to Fingerprint APIs are often blocked by ad-blockers or privacy-focused browsers like Brave. Make sure to disable your ad blocker when trying out the snippets above.
We offer a variety of integration options that allow you to protect the JS agent from ad blockers by proxying requests through your own domain. See Custom subdomain setup to get the minimum recommended ad blocker protection.
To identify 100% of visitors with or without ad blockers, we recommend using one of our cloud proxy integrations. See Protecting the JavaScript agent from ad blockers for more details.
Server API and Smart Signals
You can query identification events or visitor information using the Server API. You can retrieve an individual event by its requestId
or a visitor's entire event history by their visitorId
. JS Agent gives you both those values when it identifies a visitor.
You must authenticate your requests to the Server API using your Secret API Key. You can create one in the same place as the public API Key: Dashboard > App Settings > API Keys.
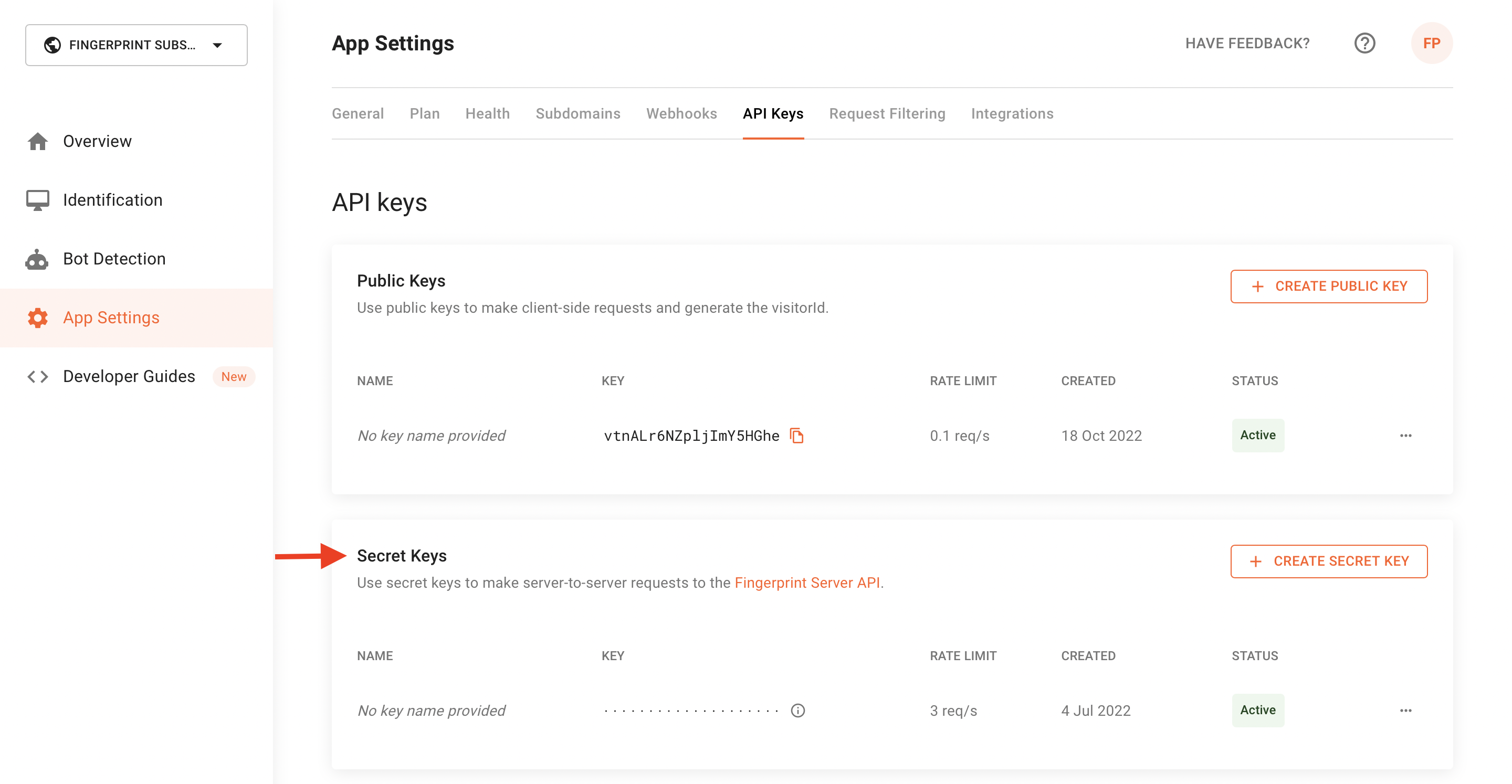
We support several server-side SDKs to help you work with the Server API in your favorite programming language. The easiest way to use our server-side SDKs is by going to App settings > Integrations > Server Libraries and picking your preferred language.
See SDKs and Libraries for more details. To receive identification events in your server application automatically as they happen, see Webhooks.
Smart signals
Server API and Webhooks allows you to access additional actionable insights about your visitors called Smart Signals. These include IP Geolocation, Bot detection, Incognito mode detection, VPN detection, Browser tampering detection, and much more. See Smart Signals Overview for more details.
Try the JS agent in your browser
Use the code editors below to try the JS agent with your credentials.
Updated about 1 month ago